Add Dynamic Watermark to Images on Your Website Using JavaScript
Adding a Dynamic Watermark to Every Images on Your Website Using JS
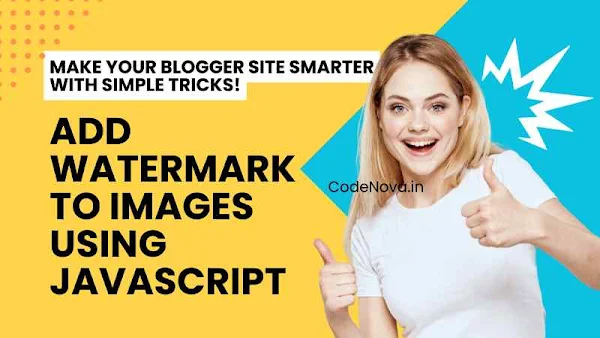
In this blog post, we’ll show you how to add a dynamic watermark to every image on your website using JavaScript. Whether you’re a blogger, website owner, or content creator, this technique is a great way to protect your images while maintaining the aesthetic of your site.
Images play a crucial role in making your website attractive, but they can also be vulnerable to unauthorized use. Adding a watermark helps prevent misuse by marking your content as copyrighted. With JavaScript, you can easily add dynamic watermarks to all images on your site without needing to modify each image manually.
What is a Dynamic Watermark?
A dynamic watermark is a transparent text or image that appears over the original image. It’s added using code rather than being embedded into the image file itself. This means the watermark can be applied to every image automatically, without changing the original image. Dynamic watermarks are particularly useful for websites that regularly update their content or have a large number of images.
Why Add a Watermark to Your Images?
There are several reasons why adding a watermark to your images is important:
- Protect Your Content: Watermarks help protect your images from being stolen or used without permission.
- Branding: A watermark can help reinforce your brand identity, making it clear that the image belongs to you.
- Copyright Protection: It signals that the image is copyrighted and can’t be reused freely.
How to Add a Dynamic Watermark Using JavaScript
Now, let’see how you can add a dynamic watermark to every image on your website using JavaScript. The process involves using the `<canvas>` element in HTML5, which allows us to overlay text or an image on top of other content, like an image.
Add JavaScript for Watermarking
Next, we’ll use JavaScript to add the watermark dynamically to the images. The JavaScript will create a `<canvas>` element, draw the image onto the canvas, and then add the watermark text on top.
// JavaScript code to add watermark
document.addEventListener("DOMContentLoaded", function () {
const watermarkText = "© codenova.in"; // Your watermark text
const images = document.querySelectorAll("img"); // Select all images
images.forEach((img) => {
const canvas = document.createElement("canvas");
const ctx = canvas.getContext("2d");
// Set canvas size to match the image
canvas.width = img.width;
canvas.height = img.height;
// Draw the original image onto the canvas
ctx.drawImage(img, 0, 0);
// Add watermark text
ctx.font = "bold 24px Arial"; // Customize font and size
ctx.fillStyle = "rgba(255, 255, 255, 0.5)"; // Semi-transparent white
ctx.textAlign = "right";
ctx.textBaseline = "bottom";
ctx.fillText(watermarkText, canvas.width - 10, canvas.height - 10); // Bottom-right corner
// Replace the original image with the canvas
img.src = canvas.toDataURL("image/png");
});
});
Step 3: Customize Your Watermark
The watermark text can be customized to suit your branding. You can change the font, size, color, and transparency. Additionally, you can adjust the watermark's position by modifying the coordinates passed to the fillText()
method.
In the code above, the watermark is added at the center of the image, but you can adjust this by changing the ctx.fillText()
position or even adding an image as a watermark instead of text.
Step 4: Handling Different Image Sizes
Different images may have different sizes, and you might want to ensure that the watermark looks good on all of them. You can scale the watermark’s size depending on the image’s dimensions.
// Add watermark text
ctx.font = "bold 24px Arial"; // Customize font and size
ctx.fillStyle = "rgba(255, 255, 255, 0.5)"; // Semi-transparent white
ctx.textAlign = "right";
ctx.textBaseline = "bottom";
ctx.fillText(watermarkText, canvas.width - 10, canvas.height - 10); // Bottom-right corner
Step 5: Add Watermark to Multiple Images
If you want to apply the watermark to multiple images on your website, you can loop through all the images with a specific class and apply the watermark to each one. The JavaScript we provided earlier automatically applies the watermark to every image with the class .watermarked-image
.
Advantages of Using JavaScript for Watermarks
Using JavaScript to add watermarks offers several advantages:
- Dynamic and Customizable: You can easily change the watermark text, size, position, and transparency without modifying the image itself.
- Non-Intrusive: Unlike static watermarks, which require image manipulation, JavaScript-based watermarks do not alter the original image.
- Easy to Implement: The code is easy to add to any website and doesn’t require complex setups or external software.
Adding a dynamic watermark to every image on your website using JavaScript is an easy and effective way to protect your content. With just a few lines of code, you can ensure that your images are marked with your brand, preventing misuse and enhancing your online presence. Whether you’re a blogger, photographer, or business owner, watermarks are a great way to safeguard your intellectual property.