SwiftUI List Section: Add Rounded Corners & Borders Easily (Full Tutorial)
Full Tutorial Rounded Corners and Border for a SwiftUI List Section
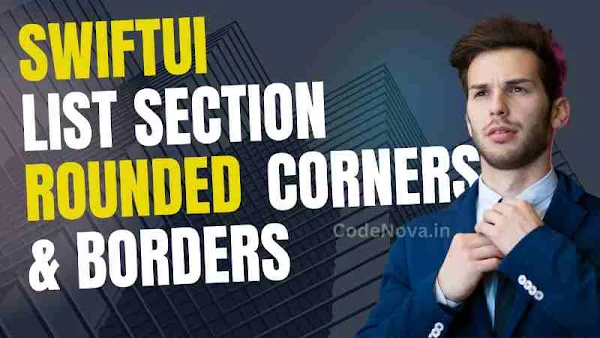
In this tutorial, we’ll explore how to add rounded corners and borders to a SwiftUI `List` section. SwiftUI has revolutionized app development with its declarative syntax, but customizing list sections requires some extra effort. This post will guide you step-by-step to create visually appealing list designs using rounded corners and borders.
SwiftUI’s `List` is a powerful component for creating scrollable content, but achieving specific visual effects like rounded corners isn’t straightforward. In this post, we’ll learn how to overcome these challenges with practical code examples and tips.
What is a SwiftUI List?
The `List` in SwiftUI is a container for presenting rows of data in a scrollable view. It can be used for static content or dynamic data from a collection. While the default `List` is functional, you may want to customize its appearance to better align with your app’s design language. Adding rounded corners and borders is one way to achieve this.
Why Add Rounded Corners and Borders?
Setting Up Your SwiftUI Project
- Step 1: Open Xcode and create a new SwiftUI project.
- Step 2: In your ContentView.swift file, set up a basic `List` with sections.
- Step 3: Run the project on the simulator to see the default `List` view.
import SwiftUI
struct ContentView: View {
var body: some View {
List {
Section(header: Text("Fruits")) {
Text("Apple")
Text("Banana")
Text("Cherry")
}
Section(header: Text("Vegetables")) {
Text("Carrot")
Text("Broccoli")
Text("Spinach")
}
}
}
}
Adding Rounded Corners to List Sections
By default, SwiftUI doesn’t provide an easy way to add rounded corners to list sections. However, you can achieve this by wrapping your section content in a custom `background` view and applying a `cornerRadius` modifier.
struct ContentView: View {
var body: some View {
List {
Section(header: Text("Fruits")) {
Text("Apple")
Text("Banana")
Text("Cherry")
}
.listRowBackground(
RoundedRectangle(cornerRadius: 10)
.fill(Color.white)
.shadow(radius: 3)
)
}
.listStyle(InsetGroupedListStyle())
}
}
This code adds rounded corners to the section’s background. The `listRowBackground` modifier allows customization of each row’s appearance.
Adding Borders to List Sections
To add borders, you can modify the background view to include a stroke around the rounded rectangle.
struct ContentView: View {
var body: some View {
List {
Section(header: Text("Fruits")) {
Text("Apple")
Text("Banana")
Text("Cherry")
}
.listRowBackground(
RoundedRectangle(cornerRadius: 10)
.stroke(Color.blue, lineWidth: 2)
.background(Color.white)
)
}
.listStyle(InsetGroupedListStyle())
}
}
This creates a bordered effect for your list section. You can customize the `Color` and `lineWidth` to match your app’s theme.
Customizing Padding and Margins
To add spacing around your list sections, you can apply padding to the rows. This ensures that the rounded corners and borders don’t feel cramped.
struct ContentView: View {
var body: some View {
List {
Section(header: Text("Fruits")) {
Text("Apple")
Text("Banana")
Text("Cherry")
}
.listRowInsets(EdgeInsets(top: 10, leading: 10, bottom: 10, trailing: 10))
.listRowBackground(
RoundedRectangle(cornerRadius: 10)
.fill(Color.white)
.shadow(radius: 3)
)
}
.listStyle(InsetGroupedListStyle())
}
}
The `listRowInsets` modifier adjusts the spacing inside the rows, enhancing the overall layout.
Using Custom Views for List Section Backgrounds
If you want even more control, you can use a custom view as the background for each section. For example:
struct SectionBackground: View {
var body: some View {
RoundedRectangle(cornerRadius: 10)
.fill(Color.white)
.shadow(radius: 3)
}
}
struct ContentView: View {
var body: some View {
List {
Section(header: Text("Fruits")) {
Text("Apple")
Text("Banana")
Text("Cherry")
}
.listRowBackground(SectionBackground())
}
.listStyle(InsetGroupedListStyle())
}
}
This approach provides flexibility for reusing custom backgrounds across multiple sections.
By adding rounded corners and borders to your SwiftUI `List` sections, you can significantly improve the visual appeal of your app. With the techniques shared in this tutorial, you now have the tools to create clean and modern designs in SwiftUI.
Experiment with different styles, colors, and shadows to make your app stand out. Happy coding!